Last updated on March 9, 2023
In WWDC 2021, Apple introduce additional features for List
further improving the component itself.
You may want to check out these new features on how it will affect your List
and that these additional features are only available on iOS 15 and above.
Prerequisites
To follow along this tutorial, you’ll need some basic knowledge in:
- A basic familiarity with Swift.
- At least Xcode 13
- iOS 15+
Bind
You can create a List
directly from binding where it’s closure allows individual binding to each element shown. With this, each row will be bind to its data.
Using this method will allow you to modify your List
without reloading the entire view when a single item changes.
This is example scenario where a list of settings is available for your app.
struct Settings: Identifiable {
let id = UUID()
var label: String
var isToggled = false
}
Based on the user’s choice, you will need to always render accordingly to what the user toggled on their setting.
@State private var settings = [
Settings(label: "Airplane Mode", isToggled: true),
Settings(label: "Dark Mode"),
Settings(label: "Bluetooth")
]
var body: some View {
List($settings) { $settings in
Text(settings.label)
Spacer()
Toggle("Toggled", isOn: $settings.isToggled)
.labelsHidden()
}
}

Separator
Doesn’t it annoy you that the fact that you need to hide the separator is so complex.
You should be glad that it’s an easier process now with this new update. By using listRowSeparator(.hidden)
, you can essentially hide the separator for the List
. As easy as that.
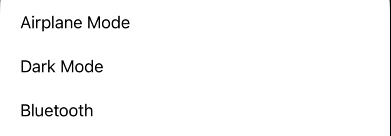
Or even change the color of the separator using .listRowSeparatorTint(.green)

Badge
With badge, you can add a text/integer next to the Text
. This could be useful to introduce new setting that is added to creata an extra attention.
Here, I’ve shown Airplane Mode as New.

Text(settings.label)
.badge($settings.isToggled.wrappedValue ? "NEW" : "")
Spacer()
Toggle("Toggled", isOn: $settings.isToggled)
.labelsHidden()
Where to go From Here
SwiftUI definitely has grown a lot in the past few years. In my point of my view, SwiftUI should be adopted in your project if your project is using iOS 13 as the minimum operating system.
Feel free to check out other posts where you can learn more about what SwiftUI is capable of.
Comments are closed.